Lets start from the beginning. You can either create an empty web api project or by creating asp .net wep application and then adding web api's to it. That's completely depend on your and project style. Sometimes you may want to add api's to existing project. So in this kind of situation you have to add web api's configurations to it. Anyway to develop asp .net web api it won't be an issue.
Lets learn this is easy way first.First we should know how this works. Then it will be easy to add manually.
Lets Start.
Go To File -> New Project.
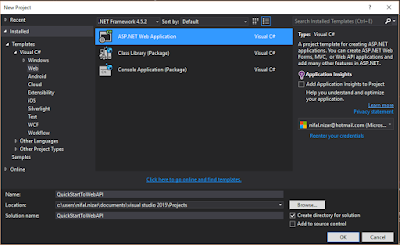
If Empty Web API Project, Then
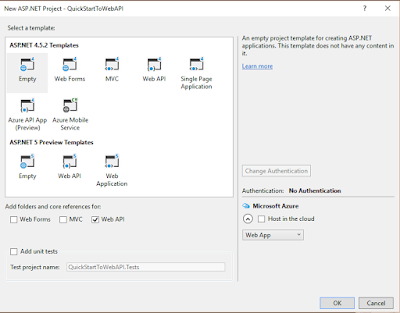
Or Else MVC Web API Project
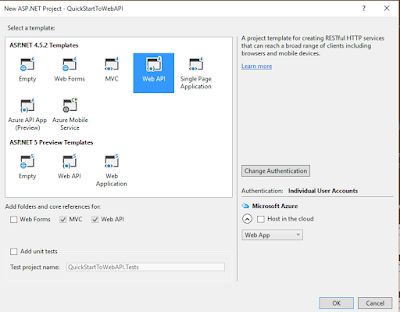
In My Example I Have Created An Empty Web API Project. My Solution Explorer Looks Like Below.
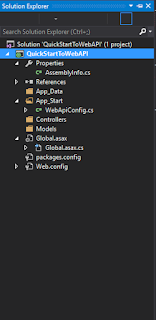
Lets Add A Controller To Our Project. Right Click Controller Folder In Solution Explorer.
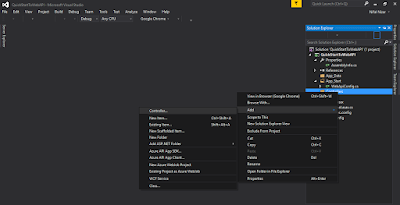
Then You Will Get Window Like Below.
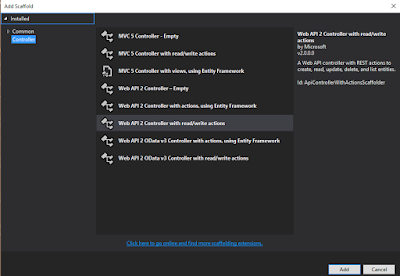
No Need to Go To Deep First. Select "Web API 2 Controller with read/write actions". This Will Give You Necessary Methods. After You Click "Add", It Will Ask You To Give A Name For Your Controller. Give What Ever Name You Want. But Make Sure It;'s Ends With Word "Controller". I'm Giving As "DefaultController", Which Automatically Comes. After Giving A Name To Controller Press "Add". You Will Get Your Controller Just Like Below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 | using System; using System.Collections.Generic; using System.Linq; using System.Net; using System.Net.Http; using System.Web.Http; namespace QuickStartToWebAPI.Controllers { public class DefaultController : ApiController { // GET: api/Default public IEnumerable<string> Get() { return new string[] { "value1", "value2" }; } // GET: api/Default/5 public string Get(int id) { return "value"; } // POST: api/Default public void Post([FromBody]string value) { } // PUT: api/Default/5 public void Put(int id, [FromBody]string value) { } // DELETE: api/Default/5 public void Delete(int id) { } } } |
You Will See By Default, Controller Has Created Five Different Methods. Lets Check Our Web API Is Working Fine. Run The Visual Studio Project. You Will See Your Browser Started A New Tab Like Below.
Don't Worry If Your Getting Something Like This. This Is Because, We Have Not Specify The Web API Yet. Go To Your Browser URL And Call Your Web API Like Below. Just After Your Domain "/api". Then Your Controller Name, But Don't Type The Controller Word Here.
http://localhost:50076/api/Default
Now If You Run You Will Get The Browser Just Like Below.
If You Are Getting This Mean You Are Successfully Calling Your Web API Method. If You Keep Break Pints In All Your Methods In Web API, U=You Will See Its Calling "Get()" Method. In Order To Call The "Get(int id)", Adjust Your URL Like Below. That Means Your Passing A Parameter To Your Web API Controller.
http://localhost:50076/api/Default/2
Hope You Had Good Start With ASP.NET Web API 2. You Can Improve Your Web API By Getting Data From Database. Rather Giving Some Default Values Like Our Example.